4
Jan.2017
Responsive Equal Height elements
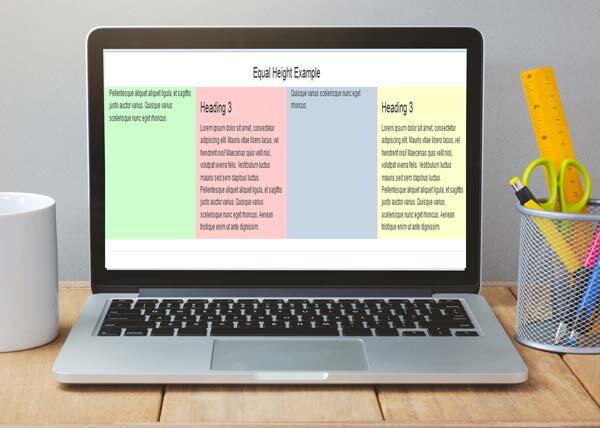
A simple JavaScript code to equalise heights of multiple elements on a page.
The basic idea is to measure all of their heights and then set all their heights to that of the tallest one.
/** * Common functionality for the theme. * * @file */ (function ($) { 'use strict'; /** * Make the same height for a group of elements. * * @param group */ $.equalHeight = function (group) { var tallest = 0; group.removeAttr('style').each(function () { var thisHeight = $(this).height(); if (thisHeight > tallest) { tallest = thisHeight; } }); group.height(tallest); }; /** * Calculate height of DIV and make the same height for each. * * @type {{attach: Function}} */ Drupal.behaviors.equalHeight = { attach: function (context, settings) { var $articlesSecondLevel1 = $('.YOUR-VIEW-CLASS', context), $articlesSecondLevel1Title = $articlesSecondLevel1.find('h3'), $articlesSecondLevel1Body = $articlesSecondLevel1.find('p'); // Make all titles and p in the same size. $.equalHeight($articlesSecondLevel1Title); $.equalHeight($articlesSecondLevel1Body); $(window).resize(function () { $.equalHeight($articlesSecondLevel1Title); $.equalHeight($articlesSecondLevel1Body); }); } }; })(jQuery);
This loops through each column's in a group and compares each element's height with thisHeight. If the current height is greater than thisHeight then thisHeight is set to tallest value.
Once the loop has finished all the group's elements are given the largest one's height.
/** * * @param root_element_prefix * @param context */ $.makeFeedbackMoreBeautiful = function (root_element_prefix, context) { $(root_element_prefix).each(function () { var $feedback_titles = $(this).find('.field-name-node-title'), $feedback_projects_about = $(this).find('.about'), $feedback_projects_wrapper = $(this).find('.project-info'), $feedback_projects_blockquotes = $(this).find('blockquote'); // Make titles the same height in the feedback section. $.equalHeight($feedback_titles); $.equalHeight($feedback_projects_about); $.equalHeight($feedback_projects_wrapper); $.equalHeight($feedback_projects_blockquotes); }); }; /** * * @param root_element_prefix */ $.revertFeedbackMoreBeautiful = function (root_element_prefix) { $(root_element_prefix).find('.field-name-node-title').height('auto'); $(root_element_prefix).find('.about').height('auto'); $(root_element_prefix).find('.project-info').height('auto'); $(root_element_prefix).find('blockquote').css('height', ''); }; /** * * @type {{attach: Drupal.behaviors.feedbackPageTweeks.attach}} */ Drupal.behaviors.feedbackPageTweeks = { attach: function (context, settings) { if ($('.view-feedback ', context).length === 0 && $('.section.feedback ', context).length === 0) { return; } if ($.getPageSize()[0] >= 768) { $.makeFeedbackMoreBeautiful('.view-feedback .row, .section.feedback', context); } $(window).resize(function () { if ($.getPageSize()[0] >= 768) { $.makeFeedbackMoreBeautiful('.view-feedback .row, .section.feedback', context); } else { $.revertFeedbackMoreBeautiful('.view-feedback .row, .section.feedback', context); } }); } };
Or another real example, if your website uses any grid like twitter bootstrap and you wish to add "equalHeight
" only for laptop ONLY and remove it for mobile devices.
If you have any suggestions, just drop a comment.
Comments